How to Represent Float Increments as Integer
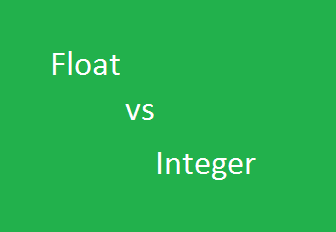
If you've always hated incrementing (or decrementing) float variables by some constant float within the range of -1 ≤ 0 ≤ 1, and increment ≠ 0, and wanted to implement a better approach by using integral data types (such as char, short, int, and long), then this article best describes the solution to your ordeal. Applications of this optimization vary from different fields of computer programming. One of which is Bersenham's Algorithm for drawing a line.
Suppose, you're adding 2 / 3 or 0.6667 to a certain float starting from 0 until it reaches a 1,000. You would write:
float fstart = 0.0f;
float fadd = 2.0f / 3.0f;
fstart = 0.0f;
do
{
fstart = fstart + fadd;
} while (fstart < 1000.0f);
Performing computations on floating-point numbers are slower compared to its integral data type counterparts of the same size. Now it gets even slower when we start casting fstart to short to be used as let's say, a coordinate in plotting a pixel.
fstart = 0.0f;
fint = 0;
do
{
fstart = fstart + fadd;
fint = (short)fstart;
} while (fstart < 1000.0f);
To convert everything to integral data types, the increment must be represented first by a fraction consisting of a numerator and a denominator. In the given case, it's 2 / 3.
Once the numerator and denominator have been determined, you need a control loop to increment a temporary variable until it is equal or it exceeds the denominator. Only by then, you increment your istart by one (1). Your temporary variable must be deducted by the denominator to preserve the increment ratio between the numerator and the denominator.
By illustration below, the ratio is preserved by each increment in the control loop.
Below is the full implementation of representing float increment by some absolute constant float in the range of of -1 ≤ 0 ≤ 1, and increment ≠ 0, using integral data type.
int t, istart, n, d;
t = 0;
istart = 0;
n = 2;
d = 3;
do
{
t += n;
if (t >= d)
{
istart++;
t -= d;
}
} while (istart < 1000);
If you can avoid any program implementation using float data types, you have to do so as it degrades the performance of application especially if the float is within a continuous loop.