How to Generate a Square Star Fractal Using Recursion
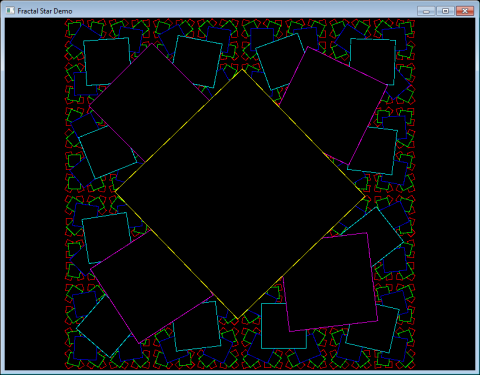
This article is about generating a Square Star Fractal using recursion (Although a triple for-loop implementation is much preferred, it will be discussed separately).
The logic of a Recursive Square Star Fractal Function is a simple Divide and Conquer algorithm. The program is subdivided into smaller tasks until it is solved. The function takes the (X, Y) middle coordinates of the square, R length, where 1 Side of Square = 1 Length = 2R length, and T as the number of times to generate 2R- sized squares.
The algorithm to recursively generate the Square Star Fractal is as follows:
- If T is 0, do not generate squares
- The Top-Left Corner position of the square is (X - R, Y - R)
- The Top-Right Corner position of the square is (X + R, Y - R)
- The Lower-Left Corner position of the square is (X - R, Y + R)
- The Lower-Right Corner position of the square is (X + R, Y + R)
- Draw a middle square at position (X, Y) with 2R length of sides
- Recurse the Fractal Square Function (go to step 1) for each Corner with a shorter side of (R / 2) and a lesser Times to recur by 1 (T - 1)
Using the algorithm above, a generic function could be implemented below:
void fractal(float x, float y, float r, int t)
{
if (t > 0)
{
fractalr(x - r, y - r, r / 2, t - 1);
fractalr(x + r, y - r, r / 2, t - 1);
fractalr(x + r, y + r, r / 2, t - 1);
fractalr(x - r, y + r, r / 2, t - 1);
drawRectangle(x - r, y - r, x + r, y + r);
}
}
Below is a sample output of a Square Star Fractal in C++ using SFML 2.5 / OpenGL with colors.
Below is a sample output of a Rotating Square Star Fractal in C++ using SFML 2.5 / OpenGL with colors.
Since the algorithm is simple for Square Star Fractals, you might want to challenge and amuse yourself by generating an Outlined version of the Fractal. The trick is to find the pattern.
If you tried to cheat and play around with the colors to generate the outlined fractal, you'll get something like the one below, which is not correct. The solution to this Outlined Square Star Fractal will be discussed in a separate article.
As much as I would like to include a Variable Polygonal Star Fractal in the program attachment, I'd rather leave it up to you as an exercise.
The attached demo program is written using SFML - a framework for OpenGL. If you have not downloaded the SFML library yet, please follow the instructions here.