Starting in the top left corner of a 2 x 2 grid, and only being able to move to the right and down, there are exactly 6 routes to the bottom right corner.
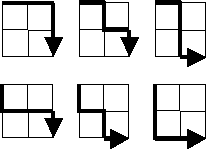
How many such routes are there through a 20 x 20 grid, or any N x N grid?
--
This is the last of the three-part series article about explaining how you could have arrived at the solution yourself in solving the Lattice Paths Problem. If you haven't tried it yet, do it first. I do not intend to deny you of your epiphany moment!
Note: All implementations are written in C/C++.
--